Q.1 What will be the output of below code?
var a;
alert(typeof(typeof a));
// string
Because typeof a store ‘’undefined’ as string.
Q.2 What will be the output of below code?
let obj = {
key:2,
value:6
};
let output = {...obj};
output.value-= obj.key;
console.log(output.value);
// 4
Q.3 What will be the output of below code?
let p = 10;
if(p === 10){
let p = 20;
}
console.log(p)
// 10
Q.4 What will be the output of below code?
const que = (x,y,z) => {
return x, y, z;
}
console.log(que(4,5,6))
//6
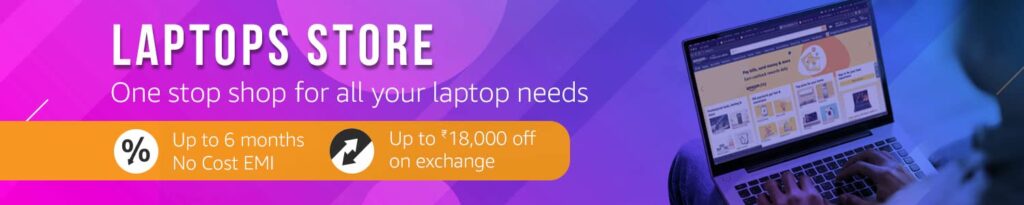
Q.5 What will be the output of below code?
let x = 6;
let y = 10;
let r = x++ + ++y - 4;
console.log(r)
// 13
Q.6 What will be the output of below code?
var name = “The Tech Genics”;
(function(){
console.log(name);
var name = “The Tech Genics”;
}
// undefined
Q.7 What will be the output of below code?
var x = 1;
if (function test (){}){
x += typeof test;
}
console.log(x);
// 1 undefined
Q.8 What will be the output of below code?
var result = '5' -2+'3';
console.log(result);
//33
Q.9 What will be the output of below code?
console.log((0.2+ 0.1) === 0.3);
//false
Q.10 What will be the output of below code?
var arr = [];
var v2 = arr.push(2);
console.log(v2)
// 1