Introduction
As you know angular team has released the version 17 early November which has lot of new features, and one of them is replacement for the old *ngIf structural directive with new @if syntax.
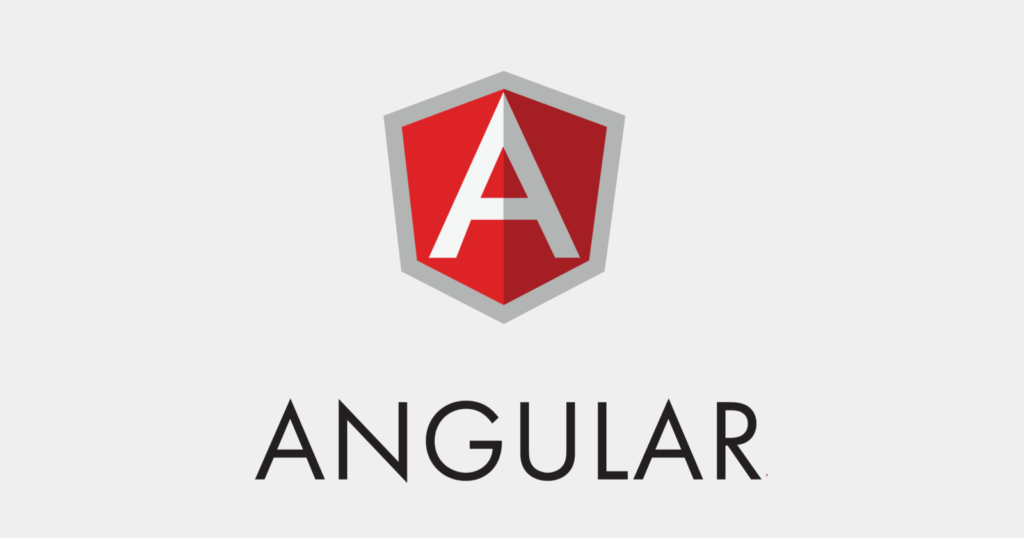
In the recent version of angular we used to have *ngIf structural directive for show/hide element into the view based on logical condition. As stated below:
<div *ngIf=”greetings”>Welcome to The Tech Genics</div>
However, Angular 17 now offers @if, a far more superior and ergonomic solution for ngIf structural directive.
What is @if in Angular 17?
@if is replacement of ngIf structural directive which can be used for rendering the element based on the logical condition. @if is more identical to the JavaScript if statement.
In comparison to the *ngIf structural directive, it is easier to read due to its clean and structured syntax. It backs the else if and else clause.
If you are using a standalone directive You don’t need to import @if it will be available by default as its part of the template engine itself, and it is not a directive.
How to use @if in Angular 17
Let’s explore how to use new syntax by writing the code.
@Component({
template: `
@if (greetings) {
<h2>Welcome to the Tech Genics!</h2>
}
`,
})
class home{
greetings: boolean = true;
}
In the above code h2 element will be rendered only when the value of greetings variable is true.
Using else if else with @if in Angular 17?
@Component({
template: `
@if (greetings) {
<h2>Welcome to the Tech Genics!</h2>
}
@else if (showRegards) {
<h2>Best Regards!</h2>
}
@else {
<h2>See you later</h2>
}
`,
})
class home{
showHello: boolean = true;
showRegards: boolean = false;
}
Benefits using @if over the *ngIf
- Due to it’s built-in support we don’t need to imports
- Supports else if and else conditions
- Not a runtime delay
- Closer to the JavaScript if syntex, this syntax is more ergonomic and verbose
- Better type checking results from increased optimal type narrowing.