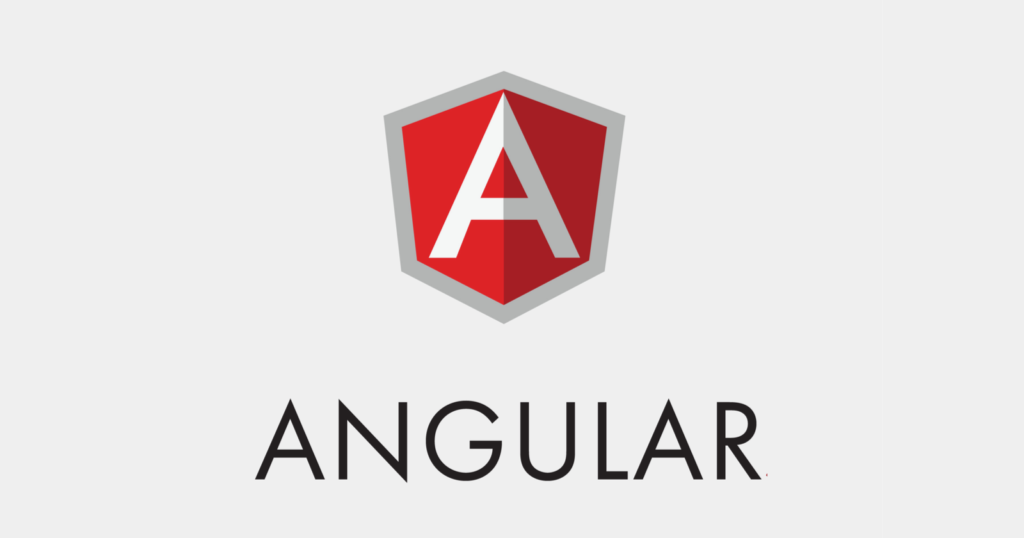
Animation is a vibrant and imaginative art style that quickly displays a sequence of still pictures, or frames, to provide the impression of motion. It is frequently used in a variety of media, including as movies, TV shows, video games, and online material. It can be 2D or 3D, manually drawn or computer-generated.
When we talk about animation on the web, we’re talking about incorporating dynamic and interactive features to improve user experience on websites and web applications. Web animation can include various techniques, such as transitions, transformations, and scrolling effects, to make web content more engaging, informative, and aesthetically pleasing. It can be used for everything from subtle hover effects on buttons to complex animated infographics and interactive storytelling. Web animation is an essential tool for web designers and developers to create visually appealing and user-friendly online experiences
Getting started with Angular Animations
@angular/animations and @angular/platform-browser are the primary Angular modules for animations
Import the core Angular functionality together with the animation-specific modules, such as @angular/animations and @angular/platform-browser, to begin adding Angular animations to your project.
As stated below:
By importing provideAnimations From @angular/platform-browser/animations we have to add it to the providers list in the bootstrapApplication call.
bootstrapApplication(AppComponent, {
providers: [
provideAnimations(),
]
});
For introducing animation capabilities we have to import BrowserAnimationsModule, into your Angular root application module.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule
],
declarations: [ ],
bootstrap: [ ]
})
export class AppModule { }
As of now we are good with importing necessary module lets learn more followed by simple example
In this example we will apply angular animation to the button which is having transition from Fade in to fade out. For example, we can specify that a button displays either Fad in or Fade out based on the user’s last action. When the button is in the Fade in state, opacity will be set to 0.5 and when it’s the Fade out opacity will be 1.
let’s have deep dive by creating new component.
Ng g component fade-in-out
If you plan to use specific animation functions in component files, import those functions from @angular/animations.
import { Component, HostBinding } from '@angular/core';
import {
trigger,
state,
style,
animate,
transition,
// ...
} from '@angular/animations';
In the next step we need to add some animation metadata property within the @component decorator and put the trigger that defines an animation within the animations metadata property.
@Component({
standalone: true,
selector: 'app-fade-in-out',
templateUrl: 'fade-in-out.component.html',
styleUrls: ['fade-in-out.component.css'],
imports: [RouterLink, RouterOutlet],
animations: [
// we'll see later on how to use trigger .
]
})
Now let’s define different state and style of the button Using Angular’s state() function, This function takes two arguments, the first argument is element state and the second is style() function.
Style() function is responsible to define set of styles to given state. You must use camelCase for style attributes that contain dashes, such as backgroundColor or wrap them in quotes, such as ‘background-color’ as mention below.
state('fade-in', style({
opacity: 0.5,
backgroundColor: 'blue'
})),
state('fade-out', style({
opacity: 1,
backgroundColor: 'blue'
}))
Style has been defined to respective state now we need to add animation to the element while travelling from one state to another. For this purpose we are going to use transition() and animate()
transition() function accepts two arguments: The first argument accepts an expression that defines the direction between two transition states, and the second argument accepts one or a series of animate() steps.
animate() function (second argument of the transition function) accepts the timings and styles input parameters. The timings parameter takes either a number or a string.As stated below:
animate (duration)
OR
animate ('duration delay easing')
We have covered everything in cut and pieces so far, but now we need to combine all the code in trigger().
The trigger() function establishes the property name to monitor for changes. When a change happens, the trigger executes the actions that were specified in its definition.
In this example, we’ll name the trigger fadeInOut, and attach it to the button element.
@Component({
standalone: true,
selector: 'app-fade-in-out',
animations: [
trigger('fadeInOut', [
state('fade-in', style({
opacity: 0.5,
backgroundColor: 'blue'
})),
state('fade-out', style({
opacity: 1,
backgroundColor: 'blue'
})),
transition('fade-in => fade-out', [
animate('1s')
]),
transition('fade-out => fade-in', [
animate('0.5s')
]),
]),
],
templateUrl: 'fade-in-out.component.html',
styleUrls: ['fade-in-out.component.css']
})
export class FadeInOutComponent {
isFadeIn = true;
toggle() {
this.isFadeIn = !this.isFadeIn;
}
}
After you’ve defined an animation trigger for a component, enclose the trigger name in brackets and precede it with a @ symbol to link it to an element in the component’s template. The trigger can then be bound to a template expression using the usual Angular property binding syntax, as demonstrated below, where expression evaluates to a defined animation state and triggerName is the name of the trigger.
The animation is executed or triggered when the expression value changes to a new state
<div [@triggerName]="expression">…</div>
The following code snippet binds the trigger to the value of the isFadeIn property.
<button type="button" (click)="toggle()">Toggle Fade In/Fade out</button>
<div [@fadeInOut]="isFadeIn ? 'fade in' : 'fade out'">
<p>The button is {{ isFadeIn ? 'Fade in' : 'Fade out' }}!</p>
</div>
Awesome! We have successfully learn how to add angular animation and style to the element.